System fonts are based on the font rendering of the corresponding operating system and offer the possibility to use the system fonts without requiring additional resources in the application.
System fonts have the advantage of usually containing all necessary characters. For example, if global online highscore lists are read, names from all over the world can appear in different languages and glyphs.
Scene Graph
The system font rendering is implemented by the corresponding platform component and is provided in the FlatTextTexture
node.
<?xml version="1.0" ?> <!-- Copyright 2013 Spraylight GmbH --> <Graph> <Namespace id="main"> <View id="view"/> <Camera id="camera" viewId="view" fieldOfViewX="400" nearPlane="400" farPlane="2500" clearColorBuffer="yes"/> <CameraTransform cameraId="camera" posX="0" posY="0" posZ="800"/> <CameraState cameraId="camera"/> <FixedParameters id="screen_parameters"/> <ParametersState parametersId="screen_parameters"/> <Reference targetId="/materials/state_plain_tex_color"/> <Transform id="position"> <FlatTextTexture id="tex_edit_text" systemFontName="TypewriterBold" fontSize="20" textColor="255i, 255i, 255i, 255i" backgroundColor="39i, 133i, 197i, 255i" text="Spraylight" textAlignmentX="CENTER" textAlignmentY="CENTER" sizeX="512" sizeY="256"/> <TextureState textureId="tex_edit_text"/> <PlaneGeometry posX="0" posY="50" frameSizeX="512" frameSizeY="256"/> <FlatTextTexture id="tex_info_text" systemFontName="SansBold" fontSize="24" textColor="29i, 29i, 27i, 255i" backgroundColor="225i, 200i, 63i, 255i" textAlignmentX="LEFT" textAlignmentY="CENTER" sizeX="256" sizeY="64"/> <TextureState textureId="tex_info_text"/> <PlaneGeometry posX="0" posY="-150" frameSizeX="256" frameSizeY="64"/> </Transform> </Namespace> </Graph>
Text Texture Definition
The FlatTextTexture
node implements a texture which is bound to the graphic hardware and has to be conform to the "power of 2" concept. In our case, the size of the first texture is set to 512x256 pixels by using the attributes sizeX="512"
and sizeY="256"
.
Font type and font size are specified with the attributes systemFontName="TypewriterBold"
and fontSize="24"
.
The font color is specified with the attribute textColor="…"
and the background color of the complete texture with backgroundColor="…"
.
For the horizontal text alignment (textAlignmentX
) the following values are available:
"CENTER"
centered in the middle of the texture (default setting)"LEFT"
aligned to the left margin of the texture"RIGHT"
aligned to the right margin of the texture
For the vertical text alignment (textAlignmentY
) the following values are available:
"CENTER"
centered in the middle of the texture (default setting)"TOP"
aligned to the upper margin of the texture"BOTTOM"
aligned to the lower margin of the texture
It is possible to specify the text to be shown with the attribute text="…"
directly in the definition of the graph or to change it afterwards in the program code.
The FlatTextTexture
can only be used, if the platform component supports system font rendering.
At the moment, all provided platforms support the following system fonts:
systemFontName="TypewriterRegular"
systemFontName="SansRegular"
systemFontName="TypewriterBold"
systemFontName="SansBold"
Texture Display
A TextureState
node needs to point at the text texture and the PlaneGeometry
node renders the texture with the corresponding parameters.
In our example, the PlaneGeometry
attributes frameSizeX="512"
and frameSizeY="256"
are set according to the dimensions of the text texture in order to achieve a 1:1 image.
Application
The system font application provides a simple function to edit a text and to display information.
Logic Initialization
Bool App::SystemFontLogic::OnInit(const Logic::IState* state) { Graph::IRoot* root = state->GetGraphRoot(); if (state->GetPlatformConfiguration()->IsTargetClassMatching(IEnums::TARGET_CLASS_HANDHELD)) { Logic::TransformNode position; position.GetReference(root, "/main/position"); if (position.IsValid()) { position->SetPositionY(200); } } AddGraphNode(mEditTextNode.GetReference(root, "/main/tex_edit_text")); AddGraphNode(mInfoTextNode.GetReference(root, "/main/tex_info_text")); if (!AreGraphNodesValid()) { return false; } mEditText = mEditTextNode->GetText(); UpdateText(); return true; }
In order to make room for the on-screen keyboard on mobile devices, the position of all text texture is moved upward in the first code block.
Afterwards, the references to both of the text texture nodes are created and the processor base class is added.
The security query if (!AreGraphNodesValid())
is recommended, if nodes are accessed already during the initialization. Otherwise, the application might crash, if a node were not found, but used despite.
Finally, the edit text is read from the text node and the text display is updated.
Logic Processing
void App::SystemFontLogic::OnProcessTick(const Logic::IState* state) { Logic::IDeviceHandler* deviceHandler = state->GetDeviceHandler(); if (!deviceHandler->IsKeyboardShowing()) { state->GetDeviceHandler()->ShowKeyboard(); } SInt32 action = deviceHandler->EditString(mEditText); if (action != 0) { if (action < 0) { mEditText += "\n"; } UpdateText(); } }
In the first code block we ensure that the on-screen keyboard is visible. If the keyboard is invisible, it will be displayed.
The second block uses a method from the Logic::IDeviceHandler
class in order to get a simple edit function. Only the input of new characters at the end of the text and the removal of the last character will work.
Text Display
void App::SystemFontLogic::UpdateText() { mEditTextNode->SetText(mEditText + "_"); String infoText; infoText += " " + Util::UInt32ToString(mEditText.GetLengthUTF8()); infoText += " Characters\n"; infoText += " " + Util::UInt32ToString(mEditText.GetLength()); infoText += " Bytes"; mInfoTextNode->SetText(infoText); }
This auxiliary function shows the edited text with an attached underscore.
The last block shows information about the edited text. It can be seen that all characters are saved in UTF-8 encoding and do not necessarily have to be in strict conformity with the byte length of the text, e.g. an umlaut or certain symbols.
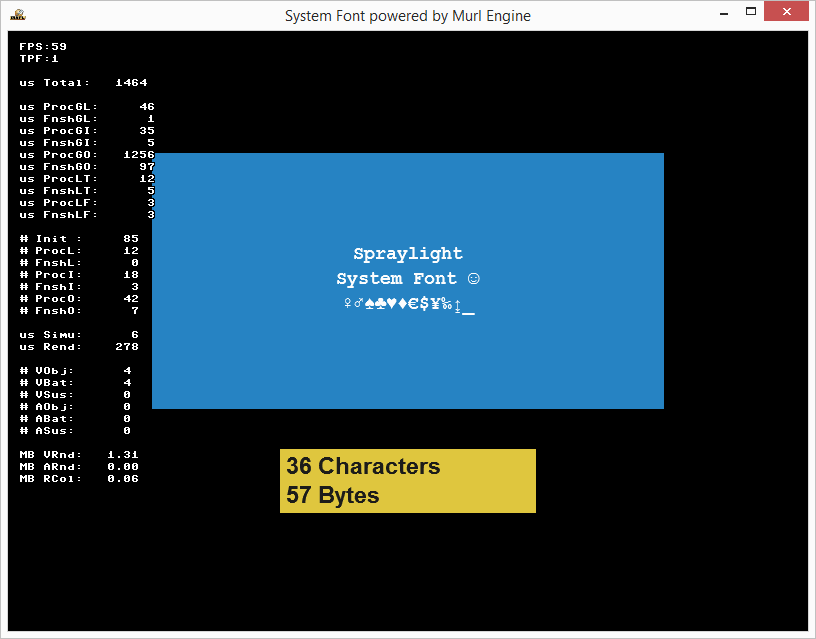